How to integrate GitHub webhooks - REST API, cURL, and Node.js
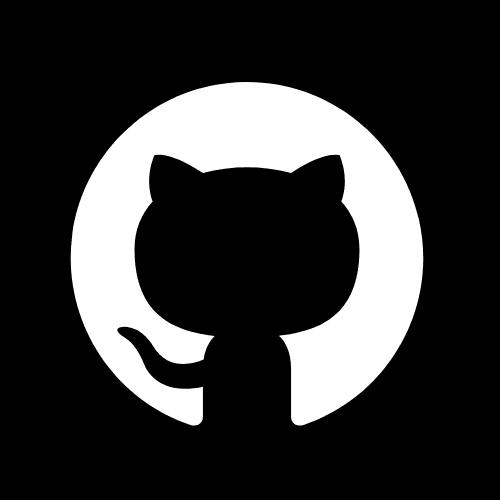
Introduction
You don't have to be an software expert to integrate and use GitHub's webhooks. I've been a software engineer for the past 4 years and have had my fair share of working with webhooks. They are always notoriusly hard to get right... BUT in this blog I will show you how to get GitHub's webhooks working in any of your projects.
There are two types of webhooks you can use with GitHub: organization webhooks and repository webhooks. Organization webhooks are triggered when events occur in an organization, while repository webhooks are triggered when events occur in a repository. In this blog, we will split the content into two sections to cover both types of webhooks.
Organization Webhooks Table of contents:
- Getting your personal access token
- Sending your first cURL or Node.js request to GitHub
- Testing your webhooks
- Use Syncd.dev to make your life easier (optional)
Repository Webhooks Table of contents:
- Getting your personal access token
- Sending your first cURL or Node.js request to GitHub
- Testing your webhooks
- Use Syncd.dev to make your life easier (optional)
Resources and links we will be using:
- GitHub account
- GitHub's organization REST API Docs ORG REST API
- GitHub's repository REST API Docs REPO REST API
- Ocktokit - only if you are using the REST API npm download
Unless you are registering webhooks for your personal account, you will need to be an admin or owner of the organization or repository to register a webhook.
GitHub Organization webhooks
The first type of webhooks we will be covering are organization webhooks. These webhooks are triggered when events occur in an organization. You can use organization webhooks to monitor and respond to changes in your organization, such as when a new member joins or when a repository is created.
Option 1: GUI
This is one of the easiest ways to register a webhook. You can do this by following these steps:
Step 1: Go to your organization's page in GitHub
Navigate to your organization's home page in GitHub. You can do this by clicking on your profile picture in the top right corner of the page and selecting your organization from the dropdown menu.
Step 2: Click the "Settings" tab
This should be located right underneath your organization's name and logo.

Step 3: Click the "Webhooks"
This should be on the left side of the page located under the "Code, planning, and automation" tab.
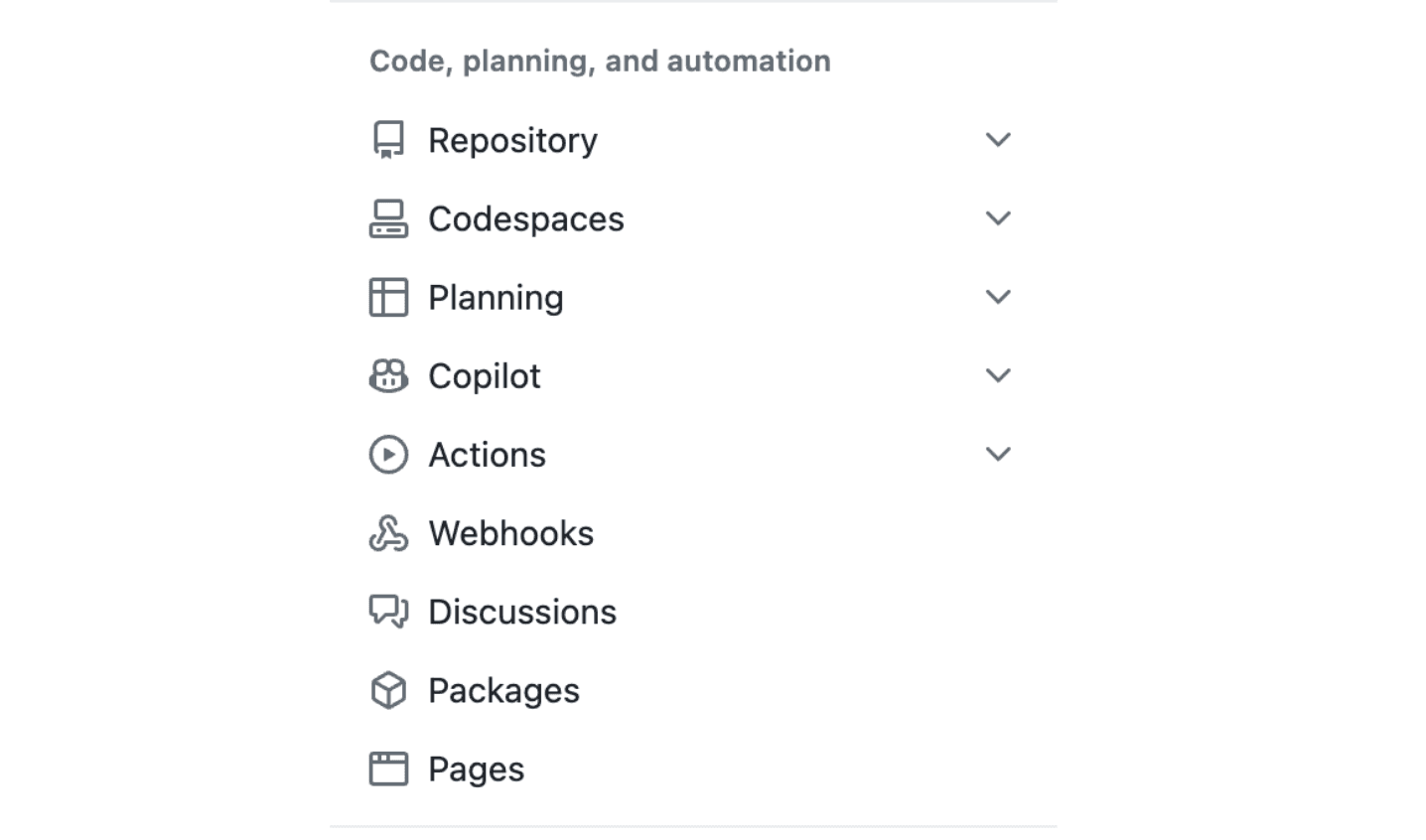
Step 4: Click "Add webhook"

After you click the "Add webhook" you will be prompted to fill out a form with the following fields:
- Payload URL
- Content type
- Secret
- Which events would you like to trigger this webhook?
- Active (checkbox) - This will enable or disable the webhook
Step 5: That's it!
You have successfully registered a webhook for your organization. You can now test it by making changes to your organization and seeing if the webhook is triggered.
Option 2: cURL request
Step 1: Get your personal access token
You will need a personal access token to authenticate your requests to GitHub's REST API. You can create a personal access token by following these steps:
- Go to your GitHub settings
- Click on the "Developer settings" tab
- Click on the "Personal access tokens" tab
- Click the "Generate new token" button
- Fill out the form with the following scopes:
- admin:org_hook
- read:org_hook
- write:org_hook
- Click the "Generate token" button
- Copy the token and save it in a safe place
Step 2: Send your first cURL request to GitHub
Now that you have your personal access token, you can send your first cURL request to GitHub's REST API to register a webhook for your organization. You can do this by following these steps:
- Open your terminal
- Run the following cURL command, replacing
YOUR-TOKEN
with the token we just generated in the previous step:
curl -L \
-X POST \
-H "Accept: application/vnd.github+json" \
-H "Authorization: Bearer <YOUR-TOKEN>" \
-H "X-GitHub-Api-Version: 2022-11-28" \
https://api.github.com/orgs/ORG/hooks \
-d '{"name":"web","active":true,"events":["push","pull_request"],"config":{"url":"http://example.com/webhook","content_type":"json"}}'
Option 3: REST API - Ocktokit
This is a pretty simple way to register a webhook. You can do this by following these steps:
- Install the Ocktokit package by running the following command in your terminal:
pnpm install octokit
- Find the personal access token from above. We will use it to authenticate your requests to GitHub's REST API.
- Here is the code to register a webhook for your organization:
await octokit.request("POST /orgs/{org}/hooks", {
org: ORG_NAME,
name: "web", // this has to be set to "web" per the docs
active: true, // if you want the endpoint to be disabled until you are ready to use it, set this to false
events: EVENTS, // these are the list of valid webhook events
config: {
url: CALLBACK_URL, // the URL that will receive the payload
secret: env.SIGNING_SECRET, // the secret used to sign the payload
insecure_ssl: 0,
content_type: "json", // you can set this to either json or form - which is application/x-www-form-urlencoded in the background
},
headers: {
"X-GitHub-Api-Version": "2022-11-28",
},
});
Option 4: Use Syncd (optional)
This is by far the easiest option, and the one we 100% recommend. All you have to do is click one button and your done. Here's how you do it with Syncd:
- Navigate to the Syncd.dev website and log in to your account.
- If you haven't already, connect your GitHub account to Syncd.dev (it's one click).
- Create a project if you haven't already (if you have skip this step and go to that project). If you have, navigate to the project you want to add the webhook to.
- Click the "+ new webhook" button.
- Choose GitHub from the dropdown.
- Fill out the form with the information you want to use.
That's it! You're done. You can now monitor, send, and debug your GitHub webhooks from the Syncd.dev dashboard.
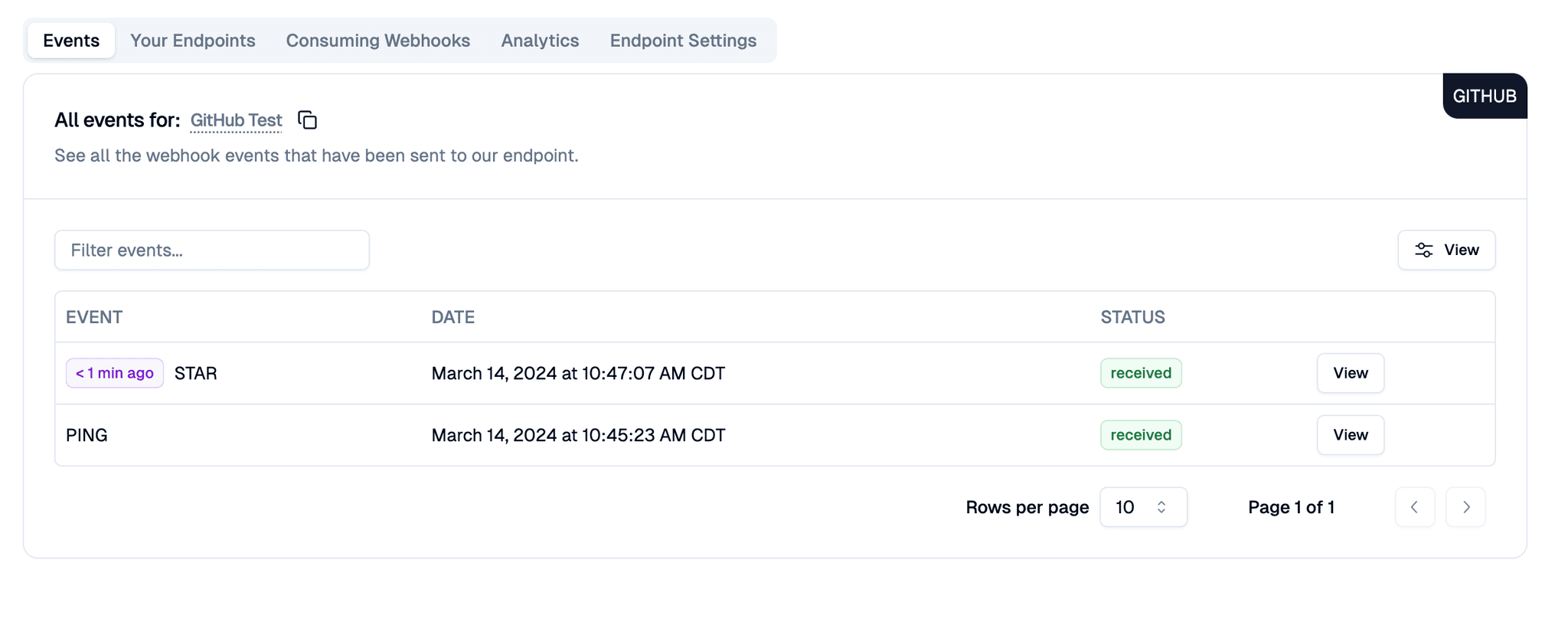
This marks the end of the GitHub organization webhooks section. If you are interested in learning how to register a webhook for a repository, please continue reading.
GitHub Repository webhooks
Option 1: GUI
Just like the GitHub organization webhook GUI option, this is one of the easiest ways to register a webhook. You can do this by following these steps:
Step 1: Go to your repository's page in GitHub
Navigate to your repository's home page in GitHub. You can do this by clicking on your profile picture in the top right corner of the page and selecting your repository from the dropdown menu.
Step 2: Click the "Settings" tab
This should be located right underneath your repository's name and logo.

Step 3: Click the "Webhooks"
This should be on the left side of the page located under the "Code, planning, and automation" tab.
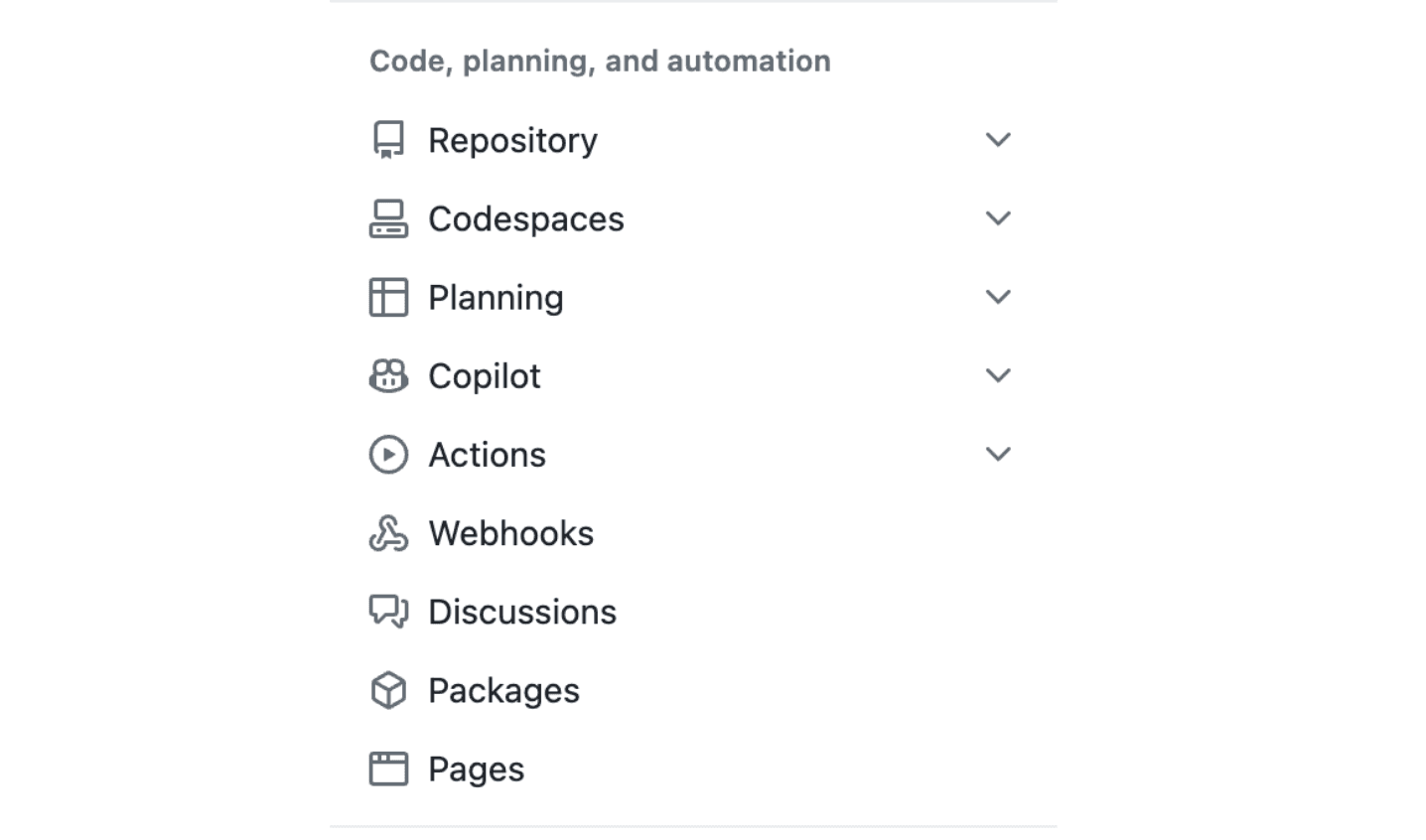
Step 4: Click "Add webhook"

After you click the "Add webhook" you will be prompted to fill out a form with the following fields:
- Payload URL
- Content type
- Secret
- Which events would you like to trigger this webhook?
- Active (checkbox) - This will enable or disable the webhook
Step 5: That's it!
Option 2: cURL request
Step 1: Get your personal access token
You will need a personal access token to authenticate your requests to GitHub's REST API. You can create a personal access token by following these steps:
- Go to your GitHub settings
- Click on the "Developer settings" tab
- Click on the "Personal access tokens" tab
- Click the "Generate new token" button
- Fill out the form with the following scopes:
- admin:org_hook
- read:org_hook
- write:org_hook
- Click the "Generate token" button
- Copy the token and save it in a safe place
Step 2: Send your first cURL request to GitHub
Now that you have your personal access token, you can send your first cURL request to GitHub's REST API to register a webhook for your organization. You can do this by following these steps:
- Open your terminal
- Run the following cURL command, replacing
YOUR-TOKEN
,OWNER
, andREPO
with your values:
curl -L \
-X POST \
-H "Accept: application/vnd.github+json" \
-H "Authorization: Bearer <YOUR-TOKEN>" \
-H "X-GitHub-Api-Version: 2022-11-28" \
https://api.github.com/repos/OWNER/REPO/hooks \
-d '{"name":"web","active":true,"events":["push","pull_request"],"config":{"url":"https://example.com/webhook","content_type":"json","insecure_ssl":"0"}}'
Option 3: REST API
This is a pretty simple way to register a webhook. You can do this by following these steps:
- Install the Ocktokit package by running the following command in your terminal:
pnpm install octokit
- Find the personal access token from above. We will use it to authenticate your requests to GitHub's REST API.
- Here is the code to register a webhook for your organization:
await octokit.request("POST /repos/{owner}/{repo}/hooks", {
owner: OWNER, // the owner of the repository could be your personal account or an organization
repo: REPO_NAME, // the name of the repository you want to register the webhook for
name: "web", // this has to be set to "web" per the docs
active: true, // if you want the endpoint to be disabled until you are ready to use it, set this to false
events: EVENTS, // these are the list of valid webhook events
config: {
url: CALLBACK_URL, // the URL that will receive the payload
secret: env.SIGNING_SECRET, // the secret used to sign the payload
insecure_ssl: 0,
content_type: "json", // you can set this to either json or form - which is application/x-www-form-urlencoded in the background
},
headers: {
"X-GitHub-Api-Version": "2022-11-28",
},
});
Option 4: Use Syncd (optional)
Once again, this is by far the easiest option, and the one we 100% recommend. All you have to do is click one button and your done. Here's how you do it with Syncd:
- Navigate to the Syncd.dev website and log in to your account.
- If you haven't already, connect your GitHub account to Syncd.dev (it's one click).
- Create a project if you haven't already (if you have skip this step and go to that project). If you have, navigate to the project you want to add the webhook to.
- Click the "+ new webhook" button.
- Choose GitHub from the dropdown.
- Fill out the form with the information you want to use.
That's it! You're done. You can now monitor, send, and debug your GitHub webhooks from the Syncd.dev dashboard.
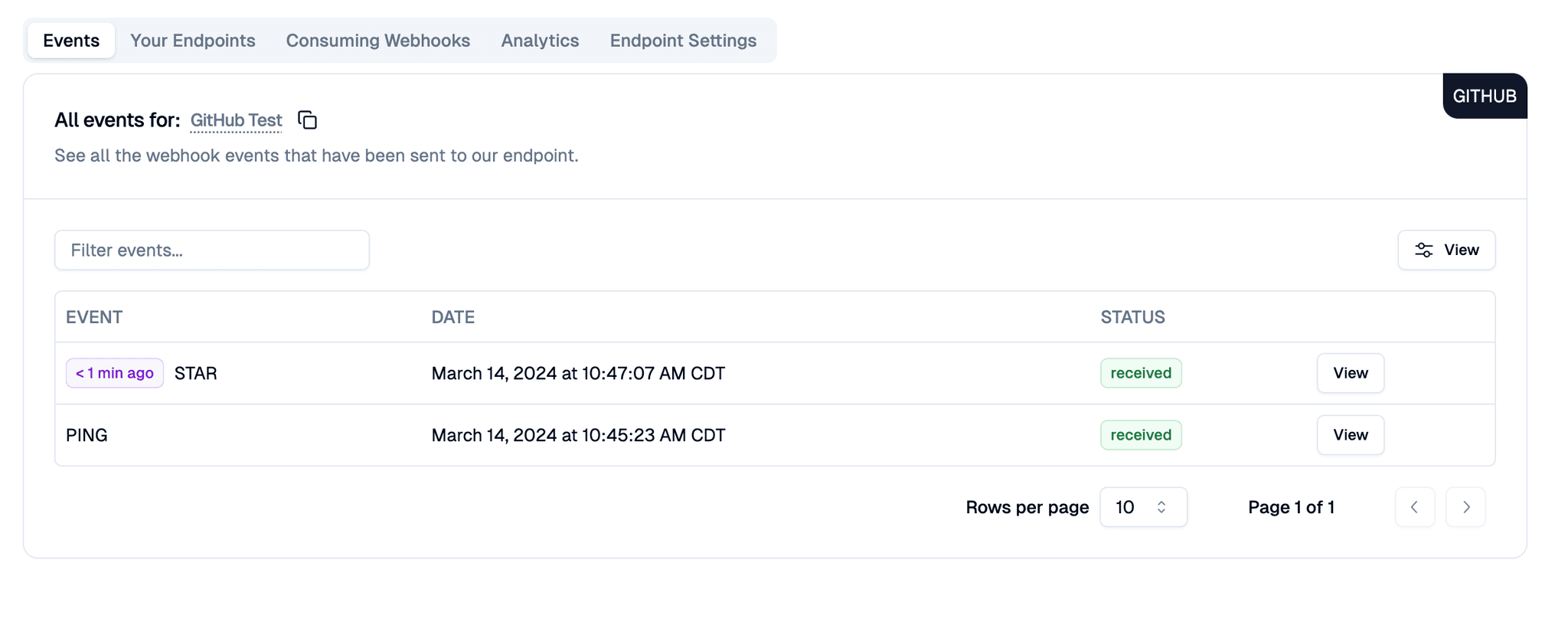
Benefits of Using Syncd.dev with GitHub's Webhooks over other Methods
Integrating github.com's webhooks through Syncd.dev offers several advantages:
- Debug and Monitor: Syncd.dev provides a centralized platform for debugging and monitoring your GitHub webhooks, allowing you to track and analyze their performance.
- Rate Limiting: Syncd.dev helps you manage rate limits and avoid overloading your GitHub account with excessive webhook requests.
- Real-Time Updates: You receive instant updates whenever changes occur in your GitHub projects, ensuring transparency across your team.
- Enhanced Collaboration: Syncd.dev allows you to trigger actions for your team members, such as notifications or automated tasks, keeping everyone on the same page.
- Streamlined Workflow: By integrating github.com's webhooks through Syncd.dev, you can automate routine tasks and streamline your development workflow, saving time and effort.
Conclusion
With Syncd.dev and github.com's webhooks, you can supercharge your development workflow and automate routine tasks effortlessly. By following the steps outlined in this blog post, you'll be able to integrate github.com's webhooks seamlessly into Syncd.dev, enabling you to stay connected and productive. Give it a try and experience the power of automation in your development process!
To learn more about Syncd.dev and its integration capabilities, visit our website at www.syncd.dev.